Top Bad Programming Practices you should Avoid
Programming is both an art and a science. However, some bad practices can hinder progress and reduce code quality. Identifying and avoiding these mistakes is crucial for efficient development and long-term success.
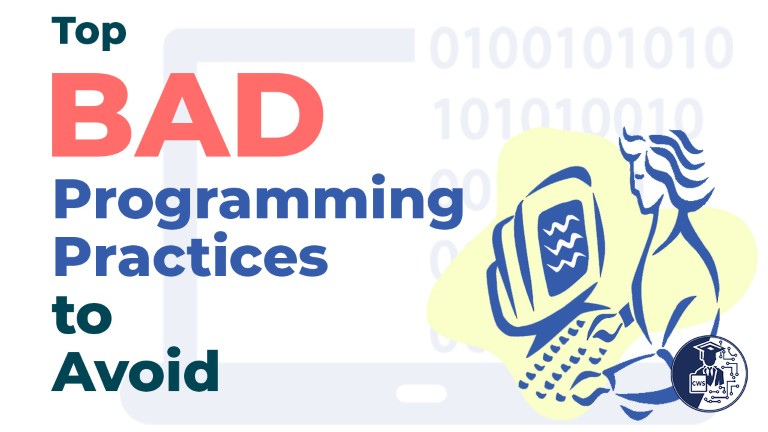
Avoiding these common mistakes is important for writing good and reliable code. Here's what you should avoid:
1. Ignoring Code Comments and Documentation
Ignoring comments makes unable to understanding code for others (Sometimes even for you). Developers should write concise, meaningful comments to explain complex logic. Clear documentation ensures smoother collaboration and future maintenance.
Why Documentation Matter?
- Helps team members understand code functionality.
- Saves time during debugging and upgrades.
- Makes teamwork easier and avoids confusion.
2. Making Code too complicated
Complex code is harder to understand, debug and maintain. Sometimes simple solution works better.
Tips to Keep Code Simple:
- Follow the KISS (Keep It Simple, Stupid) principle.
- Don’t add unnecessary features or layers.
- Simplify your code during refactoring.
3. Using confusing Function and Variables name
Using unclear or inconsistent names for variables, functions, and classes creates confusion. Good names make it clear what each part of the code does.
Best Practice for Naming:
- Use descriptive names (e.g. calculateTax instead of ct)
- Stick to one naming style, such as camelCase or snake_case.
- Avoide using abbreviations that are not obvious.
4. Using Hardcoding Values
Hardcoding values like URLs, file paths or API keys makes the code rigid and error-prone. It makes the code Hard to update later.
Alternative Approaches:
- Use configration files or environment variaables.
- Store repeated values in constant.
- Create a centralized setting system.
5. Skipping Unit Tests
Skipping tests can lead to unexpected failures in production. Unit testing ensures the reliabilty and stability of code or application.
Advantages of Testing:
- Detects bugs early in development.
- Improves confidence during deployments.
- Helps maintain consistent behaviour across updates.
6. Overusing Global Variables
Global Variables can cause unexpected problems since they are accessible everywhere in the program.
this makes debugging difficult and increases the risk of the side effects.
Solutions to minimize Globals:
- Use local variables wherever possible.
- Encapsulate data within classes or functions.
- Pass varibales where needed instead of making them global.
7. Ignoring Performance Optimization
Unoptimized code can lead to slow performance, frustrating users. Neglecting optimization often results from focusing solely on functionality.
How to optimize code:
- Use tools to find slow parts of your program.
- Simplify repeated tasks and choose better algorithms.
- Use efficient data structures, like dictionaries or trees.
8. Not handling Errors Gracefully
Ignoring potential errors or crashing on exceptions damages user experience. Proper error handling improves user experience.
Best Error Handling Practices:
- Use try-catch blocks for expected exceptions.
- Log errors with detailed messages.
- Provide meaningful feedback to users.
9. Rushing without a Plan
Diving into coding without a clear plan leads to messy, unorganized projects. A structured approach ensures better outcomes.
Steps for good Planning:
- Set clear goals and requirements.
- Break tasks into smaller tasks.
- Use version control systems like Git to manage progress.
10. Neglecting Security
Ignoring security makes your program vulnerable to hacks. Ignoring basic measures exposes applications to risks like data breaches or attacks.
Essential Security Measures:
- Check and clean user inputs to avoid attacks.
- Use encryption for sensitive data.
- Update libraries and tools to patch security issues.
Conclusion
By avoiding these mistakes, you can write clean, effective, and secure code. Always aim to improve and learn from errors to become a better programmer. Always strive for improvement and learn from mistakes to deliver top-notch software.