SOLID Principles in Programming: A guide to writting better code
The SOLID principles are five design rules that help developers write clean, maintainable, and scalable software. Introduced by Robert C. Martin (Uncle Bob), these principles ensure your code is easier to understand and modify.
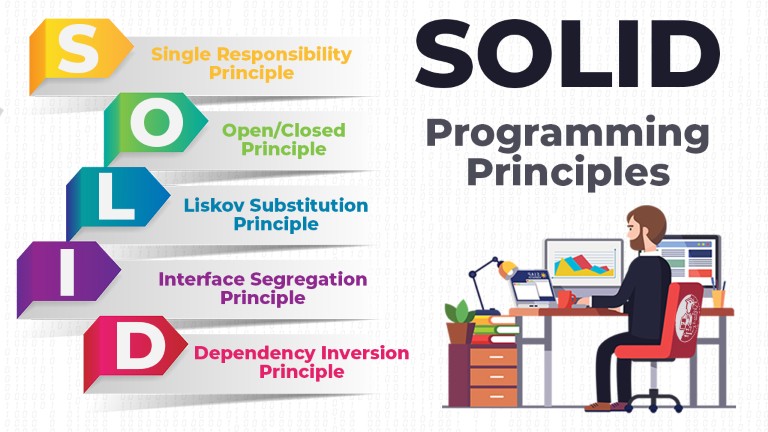
By adhering to these principles, developers can write cleaner, more efficient code. Following SOLID paves the way for robust software design, minimizing technical debt and ensuring long-term project success:
1. Single Responsibility Principle (SRP)
A class should only do one job and have one reason to change. This means each class should focus on a single responsibility.
Why SRP is important?
- Makes code easier to read and understand.
- Reduces the risk of breaking other parts when making changes.
- Simplifies future maintenance.
Example
Instead of having a User class that handles both user data and logging, separate it into User and UserLogger classes.2. Open/Closed Principle (OCP)
Software entities (classes, modules, functions) should be open for extension but closed for modification. This means you can add new functionality without altering existing code.
How to apply OCP?
- Use interfaces or abstract claees to define extensions.
- Follow inheritance and polymorphism to add functionality.
Example
Instead of modifying a PaymentProcessor class to handle new payment methods, create subclasses like CreditCardProcessor or PaypalProcessor.3. Liskov Substitution Principle (LSP)
You should be able to replace a parent class with its child class without breaking the program. A subclass must behave as the parent expects.
How to follow LSP?
- Avoid overriding methods in a way that changes their original behavior.
- Use consistent interfaces for parent and child classes.
Example
A Rectangle class and its subclass Square should both work correctly when used in the same way, provided they share the same interface.4. Interface Segregation Principle (ISP)
A class should only implement the parts of an interface it actually needs. Avoid forcing a class to use unnecessary methods.
Why ISP is useful?
- Reduces unwanted dependencies.
- Makes code easier to understand and test.
Example
Instead of one big Shape interface with methods like draw and calculateArea, split it into smaller interfaces like Drawable and AreaCalculable.5. Dependency Inversion Principle (DIP)
High-level parts of your program (main logic) should not depend on low-level parts (specific tools). Both should depend on common abstractions.
How to use DIP?
- Use dependency injection to pass required objects.
- Depend on interfaces, not specific implementations.
Example
Instead of having a Notification class directly use EmailService, make both depend on a Notifier interface.Why SOLID Principles Matter?
- Scalability: You can easily add new features without affecting existing code.
- Maintainability: Debugging and updates become simpler.
- Team Collaboration: Clear class responsibilities make it easier for teams to work together.
By following the SOLID principles, you create clean, efficient, and reliable code. These principles reduce technical debt and ensure your software is ready for future growth.